How to Automatically Restart Nginx When It Goes Down?
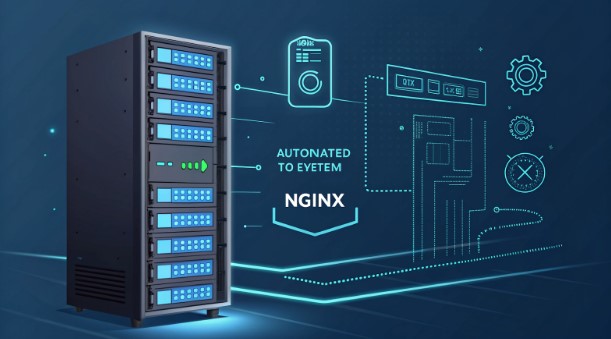
Maintaining high availability for Nginx servers is crucial for US hosting environments. When your Nginx server crashes unexpectedly, every second of downtime can impact user experience and business operations. This guide explores battle-tested methods to automatically restart Nginx when it goes down, ensuring optimal uptime for your web applications.
Understanding Why Nginx Crashes
Before diving into solutions, let’s examine common causes of Nginx failures in production environments:
- Memory exhaustion due to traffic spikes
- Configuration syntax errors after updates
- System resource limitations
- Kernel-level issues
- Unexpected process termination
Prerequisites for Automated Restart
Ensure your system meets these requirements:
- Root or sudo access to your server
- Nginx installed and configured
- Basic command line knowledge
- Text editor (vim, nano, or similar)
Method 1: Creating a Bash Monitoring Script
Let’s create a robust shell script that monitors Nginx status and triggers automatic restarts:
#!/bin/bash
# nginx_monitor.sh
CHECK_INTERVAL=60 # Check every 60 seconds
while true
do
if ! pgrep nginx >/dev/null
then
echo "$(date): Nginx is down. Attempting restart..." >> /var/log/nginx/autostart.log
systemctl start nginx
if [ $? -eq 0 ]; then
echo "$(date): Nginx restart successful" >> /var/log/nginx/autostart.log
else
echo "$(date): Nginx restart failed" >> /var/log/nginx/autostart.log
fi
fi
sleep $CHECK_INTERVAL
done
To implement this solution:
- Save the script as
/usr/local/bin/nginx_monitor.sh
- Make it executable:
chmod +x /usr/local/bin/nginx_monitor.sh
- Create a systemd service to run the script automatically
Setting Up Systemd Service for the Monitor Script
Create a systemd service file to ensure our monitoring script runs automatically on boot and restarts if it fails:
# /etc/systemd/system/nginx-monitor.service
[Unit]
Description=Nginx Monitoring Service
After=network.target nginx.service
[Service]
Type=simple
ExecStart=/usr/local/bin/nginx_monitor.sh
Restart=always
RestartSec=10
[Install]
WantedBy=multi-user.target
Enable and start the service with:
systemctl daemon-reload
systemctl enable nginx-monitor
systemctl start nginx-monitor
Method 2: Leveraging Systemd’s Built-in Recovery
Modern US hosting environments typically use systemd. We can configure it to handle Nginx crashes automatically. Modify your Nginx systemd service file:
# /etc/systemd/system/nginx.service.d/override.conf
[Service]
Restart=always
RestartSec=5s
StartLimitInterval=500s
StartLimitBurst=5
These parameters tell systemd to:
- Always restart Nginx when it crashes
- Wait 5 seconds between restart attempts
- Allow 5 restart attempts within 500 seconds
- Reset the counter after successful uptime
Method 3: Implementing Monit for Advanced Monitoring
For enterprise-grade monitoring, Monit offers sophisticated control over Nginx processes. Install Monit on your server:
apt-get update && apt-get install monit -y
Configure Monit to watch Nginx:
# /etc/monit/conf.d/nginx
check process nginx with pidfile /var/run/nginx.pid
start program = "/usr/sbin/service nginx start"
stop program = "/usr/sbin/service nginx stop"
if failed host 127.0.0.1 port 80 protocol http
then restart
if 5 restarts within 5 cycles then timeout
if memory usage > 90% for 5 cycles then restart
Best Practices for Nginx Uptime Management
Beyond automated restarts, implementing these practices will significantly improve your Nginx reliability in US hosting environments:
1. Implement Proper Logging
Configure detailed logging to track restart events and identify patterns:
# /etc/nginx/nginx.conf
error_log /var/log/nginx/error.log warn;
error_log /var/log/nginx/debug.log debug;
http {
log_format detailed '$remote_addr - $remote_user [$time_local] '
'"$request" $status $body_bytes_sent '
'"$http_referer" "$http_user_agent" '
'$request_time';
access_log /var/log/nginx/access.log detailed buffer=32k flush=5s;
}
2. Resource Monitoring Setup
Create a comprehensive monitoring script that checks multiple health indicators:
#!/bin/bash
# health_check.sh
NGINX_THRESHOLD=80
EMAIL="admin@yourdomain.com"
check_nginx_workers() {
worker_count=$(ps aux | grep "nginx: worker" | grep -v grep | wc -l)
if [ $worker_count -lt 1 ]; then
echo "Critical: No Nginx workers found" | mail -s "Nginx Alert" $EMAIL
systemctl restart nginx
fi
}
check_memory_usage() {
memory_usage=$(free | grep Mem | awk '{print $3/$2 * 100.0}')
if [ $(echo "$memory_usage > $NGINX_THRESHOLD" | bc) -eq 1 ]; then
echo "Warning: High memory usage - $memory_usage%" | mail -s "Memory Alert" $EMAIL
fi
}
check_nginx_workers
check_memory_usage
3. Load Balancing Configuration
For high-traffic scenarios, implement load balancing to distribute requests and prevent server overload:
http {
upstream backend {
server backend1.example.com:8080 max_fails=3 fail_timeout=30s;
server backend2.example.com:8080 max_fails=3 fail_timeout=30s;
keepalive 32;
}
server {
location / {
proxy_pass http://backend;
proxy_http_version 1.1;
proxy_set_header Connection "";
health_check interval=5s fails=3 passes=2;
}
}
}
Troubleshooting Common Issues
When implementing automatic restart solutions, you might encounter these challenges:
- Infinite restart loops due to configuration errors
- Resource exhaustion from rapid restart attempts
- Monitoring service conflicts
- Log rotation issues
Address these issues by:
# Add to /etc/nginx/nginx.conf
worker_rlimit_nofile 30000;
events {
worker_connections 10000;
multi_accept on;
use epoll;
}
# System limits in /etc/security/limits.conf
nginx soft nofile 30000
nginx hard nofile 30000
Advanced Configuration for Enterprise Environments
For US hosting providers handling mission-critical applications, implement these advanced configurations:
1. Zero-Downtime Reload Script
#!/bin/bash
# graceful_reload.sh
# Verify configuration before reload
nginx -t
if [ $? -ne 0 ]; then
echo "Configuration test failed. Aborting reload."
exit 1
fi
# Perform graceful reload
kill -HUP $(cat /var/run/nginx.pid)
# Verify service status
sleep 2
systemctl status nginx | grep "active (running)"
2. Enhanced Monitoring Integration
Integrate with external monitoring services using a custom health check endpoint:
location /health {
access_log off;
add_header Content-Type text/plain;
return 200 'OK';
}
3. Automated Backup Configuration
#!/bin/bash
# backup_nginx_config.sh
BACKUP_DIR="/etc/nginx/backups"
DATE=$(date +%Y%m%d_%H%M%S)
# Create backup directory
mkdir -p $BACKUP_DIR
# Backup configuration files
tar -czf $BACKUP_DIR/nginx_config_$DATE.tar.gz /etc/nginx/
# Keep only last 7 days of backups
find $BACKUP_DIR -type f -mtime +7 -delete
Conclusion and Best Practices Summary
Maintaining high availability for Nginx servers in US hosting environments requires a multi-layered approach. By implementing automated restart mechanisms, proper monitoring, and preventive measures, you can achieve optimal uptime for your web applications. Remember to:
- Test all monitoring scripts in a staging environment first
- Implement proper logging and alerting mechanisms
- Regularly review and update your monitoring thresholds
- Keep backup configurations readily available
- Document all custom solutions for team reference
Stay proactive in your nginx monitoring and automation strategy to ensure reliable hosting services and minimize downtime. Consider implementing these solutions as part of your broader server management toolkit, especially in demanding US hosting and colocation environments.