How to Build an AI Discord Bot with DeepSeek?
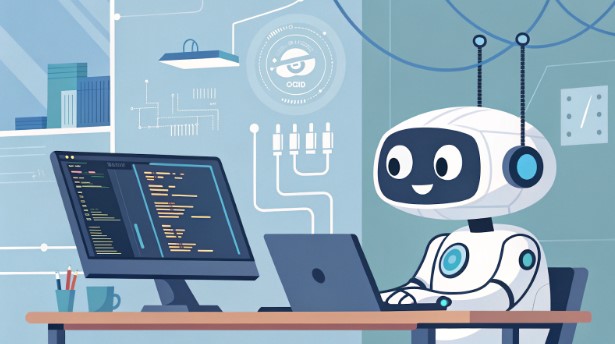
Creating an AI-powered Discord bot with DeepSeek opens up exciting possibilities for server automation and intelligent interactions. When combined with reliable Hong Kong hosting, your bot can deliver superior performance for users across Asia-Pacific. This comprehensive guide walks you through the entire process, from initial setup to deployment, with practical code examples and optimization techniques.
Prerequisites and Environment Setup
Before diving into bot development, ensure you have the following tools and configurations ready:
- Python 3.8 or higher
- Discord Developer Account
- DeepSeek API credentials
- Code editor (VS Code recommended)
First, let’s set up our project structure and install necessary dependencies:
mkdir discord-deepseek-bot
cd discord-deepseek-bot
python -m venv venv
source venv/bin/activate # For Unix
venv\Scripts\activate # For Windows
pip install discord.py python-dotenv requests
Setting Up Your Discord Bot
The first step is creating a Discord application and obtaining your bot token. Navigate to the Discord Developer Portal and follow these steps:
- Create a new application
- Go to the Bot section
- Generate and copy your bot token
- Enable necessary intents under Privileged Gateway Intents
Create a .env file in your project root to safely store your credentials:
DISCORD_TOKEN=your_discord_token_here
DEEPSEEK_API_KEY=your_deepseek_api_key
Core Bot Implementation
Here’s the basic structure of our AI-powered Discord bot. This implementation includes message handling and DeepSeek integration:
import os
import discord
from discord.ext import commands
import requests
from dotenv import load_dotenv
load_dotenv()
class AIBot(commands.Bot):
def __init__(self):
super().__init__(command_prefix='!', intents=discord.Intents.all())
self.deepseek_api_key = os.getenv('DEEPSEEK_API_KEY')
self.deepseek_endpoint = 'https://api.deepseek.com/v1/chat/completions'
async def process_ai_response(self, user_input):
headers = {
'Authorization': f'Bearer {self.deepseek_api_key}',
'Content-Type': 'application/json'
}
payload = {
'model': 'deepseek-chat',
'messages': [{'role': 'user', 'content': user_input}],
'temperature': 0.7
}
try:
response = requests.post(
self.deepseek_endpoint,
headers=headers,
json=payload
)
return response.json()['choices'][0]['message']['content']
except Exception as e:
return f"Error processing request: {str(e)}"
bot = AIBot()
@bot.event
async def on_ready():
print(f'{bot.user} has connected to Discord!')
@bot.command(name='ask')
async def ask(ctx, *, question):
async with ctx.typing():
response = await bot.process_ai_response(question)
await ctx.send(response)
bot.run(os.getenv('DISCORD_TOKEN'))
Optimizing Performance with Hong Kong Hosting
When deploying your AI Discord bot, choosing the right hosting location is crucial. Hong Kong hosting offers several advantages for APAC users:
- Low latency for Asian users
- Excellent connectivity to major tech hubs
- Reliable infrastructure
- Strategic geographic location
For optimal performance, consider these hosting configuration recommendations:
# Recommended server specifications
CPU: 2+ cores
RAM: 4GB minimum
Storage: 40GB SSD
Network: 100Mbps+
Location: Hong Kong data center
Advanced Features and Error Handling
To make your bot more robust, implement rate limiting and error handling mechanisms. Here’s an enhanced version of the message processing system:
from discord.ext import commands
import asyncio
from collections import deque
from datetime import datetime, timedelta
class RateLimiter:
def __init__(self, requests_per_minute):
self.requests = deque()
self.rpm = requests_per_minute
def can_make_request(self):
now = datetime.now()
while self.requests and now - self.requests[0] > timedelta(minutes=1):
self.requests.popleft()
if len(self.requests) < self.rpm:
self.requests.append(now)
return True
return False
class EnhancedAIBot(commands.Bot):
def __init__(self):
super().__init__(command_prefix='!')
self.rate_limiter = RateLimiter(requests_per_minute=30)
async def handle_response(self, ctx, question):
if not self.rate_limiter.can_make_request():
await ctx.send("Rate limit exceeded. Please try again later.")
return
try:
response = await self.process_ai_response(question)
return response
except Exception as e:
await ctx.send(f"An error occurred: {str(e)}")
return None
Deployment Best Practices
When deploying your bot on Hong Kong hosting infrastructure, follow these optimization strategies:
- Use process managers like PM2 for automatic restarts
- Implement logging for monitoring
- Set up SSL for secure communications
- Configure automatic backups
Here's a sample PM2 configuration file (ecosystem.config.js):
module.exports = {
apps: [{
name: "discord-bot",
script: "bot.py",
interpreter: "python3",
instances: 1,
autorestart: true,
watch: false,
max_memory_restart: "1G",
env: {
NODE_ENV: "production"
}
}]
}
Monitoring and Maintenance
Implement these monitoring practices to ensure optimal performance:
- Set up health checks
- Monitor memory usage
- Track API response times
- Implement error logging
Troubleshooting Common Issues
Here are solutions for frequently encountered problems:
- Connection timeouts: Check network configuration and firewall settings
- Memory leaks: Implement proper garbage collection
- Rate limiting: Adjust request patterns
- API errors: Verify credentials and endpoint URLs
Building an AI Discord bot with DeepSeek requires careful planning and implementation. By hosting your bot on Hong Kong servers, you can achieve optimal performance for APAC users while maintaining high availability. Remember to regularly update your bot's features and monitor its performance to ensure the best user experience.